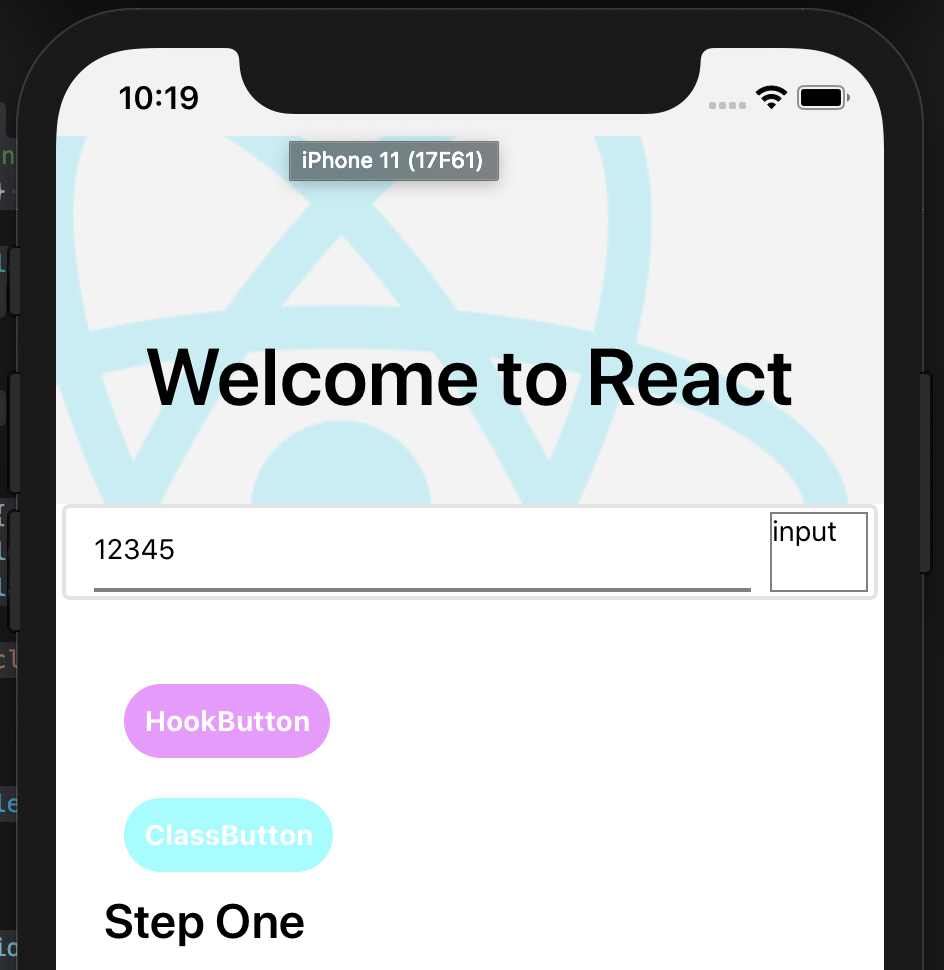
分別用Hook 與Class 建立兩個類似的 Button
<HookButton lable='HookButton'/>
<ClassButton lable='ClassButton'/>
Hooks
import React, { useState, useEffect } from 'react';
import {
StyleSheet,
Dimensions,
Text,
TouchableHighlight,
View
} from "react-native";
// 取得屏幕的宽高Dimensions
const { width, height } = Dimensions.get('window');
function HookButton(props) {
const [Lable, setLable] = useState("button");
// 相似於 componentDidMount 和 componentDidUpdate:
useEffect(() => {
// 使用瀏覽器 API 更新文件標題
if (props.lable != null) {
setLable(props.lable)
}
console.warn(`You clicked ${Lable} times`)
});
return (
<View style={styles.centeredView}>
<View
style={{
flex: 1,
flexDirection: 'row',
width: width,
}}>
<TouchableHighlight
style={styles.openButton}
onPress={() => {
props.onPress
}}
>
<Text style={styles.textStyle}>{Lable}</Text>
</TouchableHighlight>
</View>
</View>
);
}
export default HookButton;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}, openButton: {
backgroundColor: "#F194FF",
borderRadius: 20,
padding: 10,
elevation: 2,
margin: 10,
},
textStyle: {
color: "white",
fontWeight: "bold",
textAlign: "center"
},
});
Class
import React, { useState, useEffect } from 'react';
import {
StyleSheet,
Dimensions,
Text,
TouchableHighlight,
View
} from "react-native";
// 取得屏幕的宽高Dimensions
const { width, height } = Dimensions.get('window');
export default class ClassButton extends React.Component {
constructor(props) {
super(props);
this.state = {
lable: 'Button',
};
}
componentDidMount() {
if (this.props.lable != null) {
this.setState({ lable: this.props.lable })
}
console.warn(`You clicked ${this.state.Lable} times`)
}
render() {
return (
<View style={styles.centeredView}>
<View
style={{
flex: 1,
flexDirection: 'row',
width: width,
}}>
<TouchableHighlight
style={styles.openButton}
onPress={() => {
this.props.onPress
}}
>
<Text style={styles.textStyle}>{this.state.lable}</Text>
</TouchableHighlight>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}, openButton: {
backgroundColor: "#4FF9",
borderRadius: 20,
padding: 10,
elevation: 2,
margin: 10,
},
textStyle: {
color: "white",
fontWeight: "bold",
textAlign: "center"
},
});
.....誒?Hook 與Class 的 Button 可以ㄧ起使用?( º﹃º )