有了上一個部分的作業,充分的執行了前端UI的輸入,後端Server運算,與前端UI的再顯示結果功能.
本段,將就前後端往返的Action部分,進行進階的程式控制.
1. Reactivity in R
Reactiveity 流程
以前篇的例子來看,透過畫面的拉bar拉動,基本上就是使用者自UI給了新的Input,這時即透過Reactivity Notify傳遞給Server進行運算,運算完畢之後,再回應給UI進行顯示。
兩段的流程Step圖示如下:
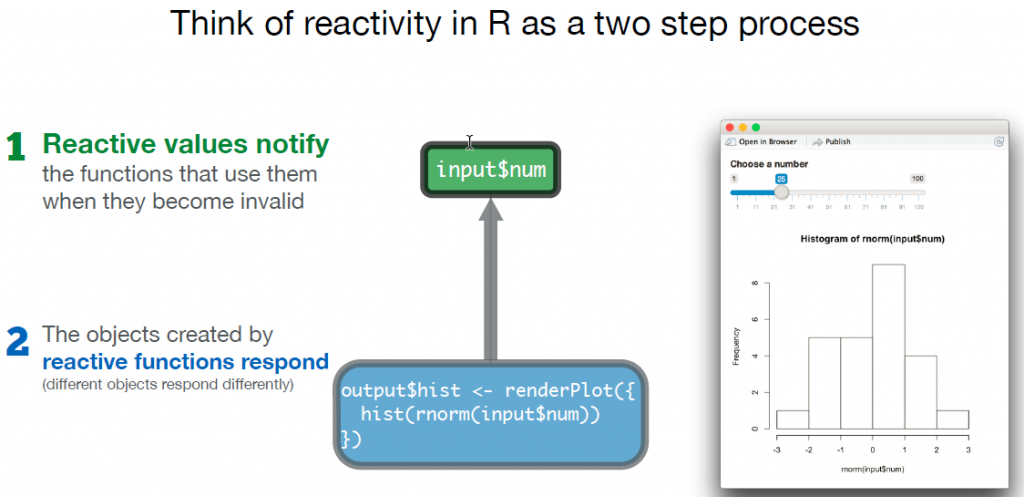
Reactiveity值傳遞
Reactiveity Values的傳遞,重點如下:
- Reactive values act as the data streams that flow through your app.
- The input list is a list of reactive values. Thevalues show the current state of the inputs.
- You can only call a reactive value from a function that is designed to work with one.
- Reactive values notify. The objects created by reactive functions respond.
基本流程(即時互動)
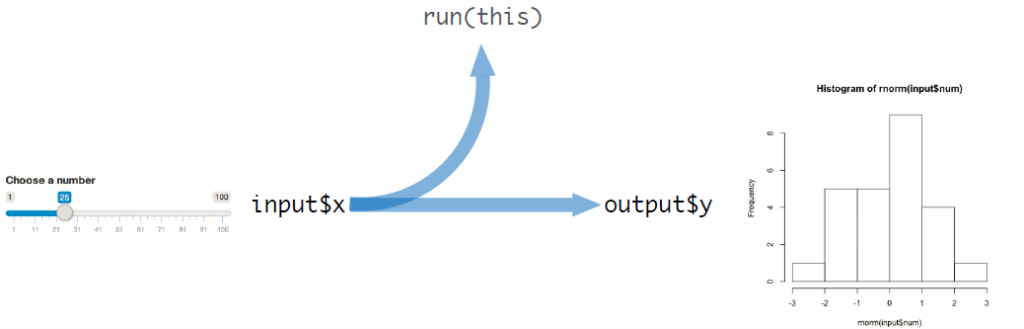
可透過處理,有不同的互動方式(即時/人工(延時)觸發)
2.reactive()函數相關模組化作業
首先先來看看這段作業
# 02-two-outputs
library(shiny)
ui <- fluidPage(
sliderInput(inputId = "num",
label = "Choose a number",
value = 25, min = 1, max = 100),
plotOutput("hist"),
verbatimTextOutput("stats")
)
server <- function(input, output) {
output$hist <- renderPlot({
hist(rnorm(input$num))
})
output$stats <- renderPrint({
summary(rnorm(input$num))
})
}
shinyApp(ui = ui, server = server)
本段程式是一個簡易的案例,可以看見一個input$num,將對應有兩組輸出:
- rutput$hist <- renderPlot
- output$stats <- renderPrint
而根據下圖的流程說明,input$num這個為共用的物件在傳遞。
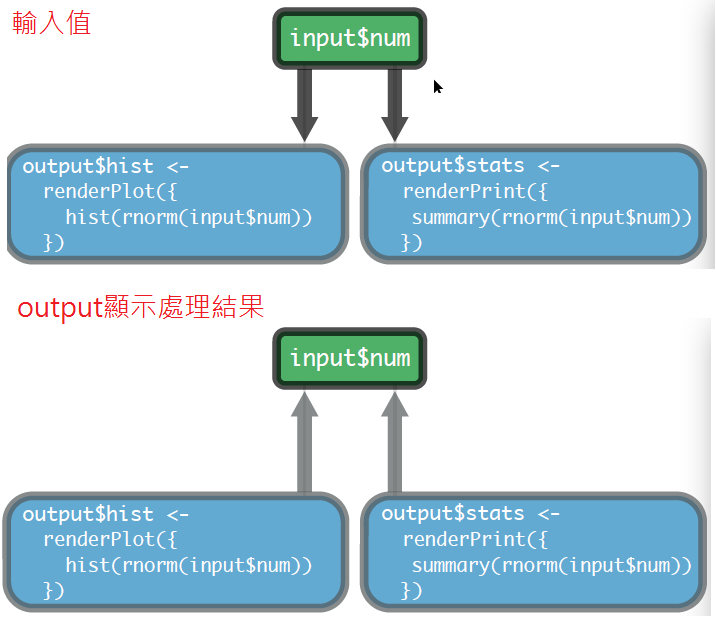
這類共用的物件,將可透過reactive()函數,將這類共用的部份抽出當作共用的模組的部分。
(例:data <- reactive({rnorm(input$num)}))
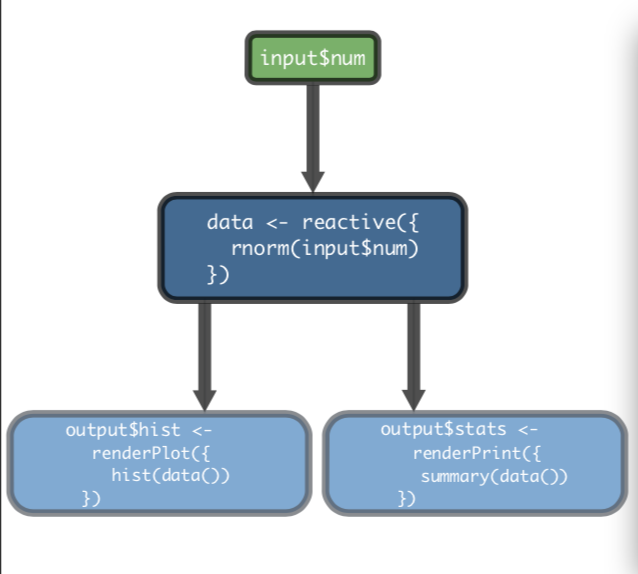
reactive() 重點提示:
- reactive() makes an object to use (indownstream code)
- Reactive expressions are themselves reactive. Use them to modularize your apps.
- Call a reactive expression like a function
- Reactive expressions cache their values to avoid unnecessary computation
2. Trigger Code
Prevent reactions with isolate()
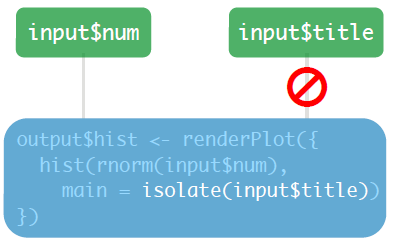
重點提示
- isolate() makes an non-reactive object
- Use isolate() to treat reactive values like normal R values
Trigger code with observeEvent() / observe()
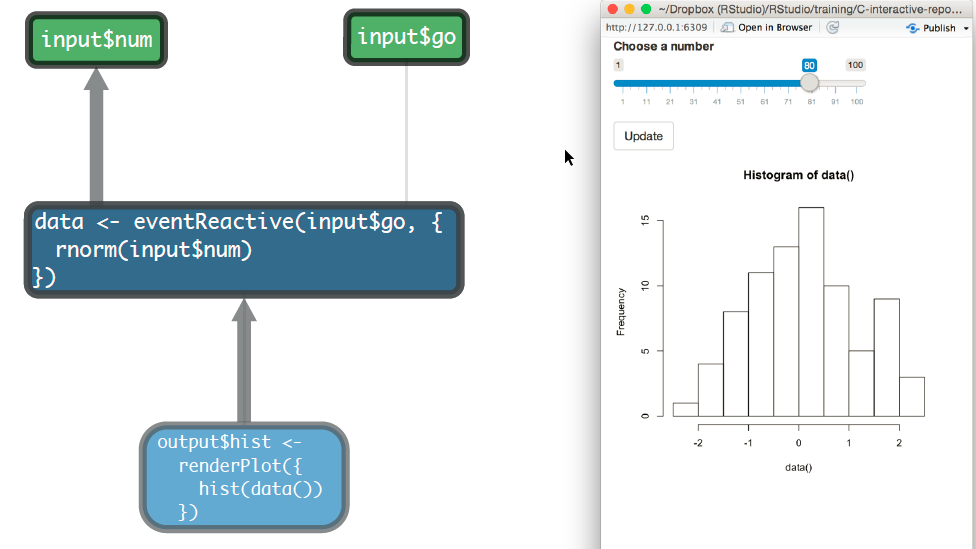
重點提示
- observeEvent() triggers code to run on the server
- Specify precisely which reactive values should invalidate the observer
- Use observe() for a more implicit syntax
Delay reactions with eventReactive()
重點提示
- Use eventReactive() to delay reactions
- eventReactive() creates a reactive expression
- You can specify precisely which reactive values should invalidate the expression
Manage state with reactiveValues()
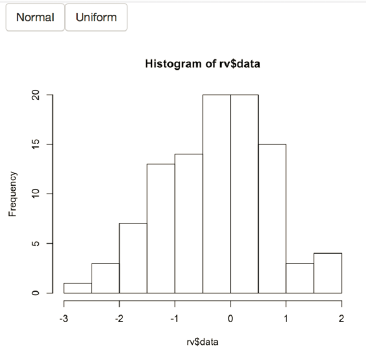
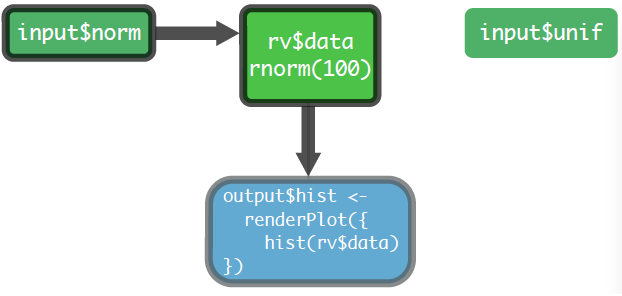
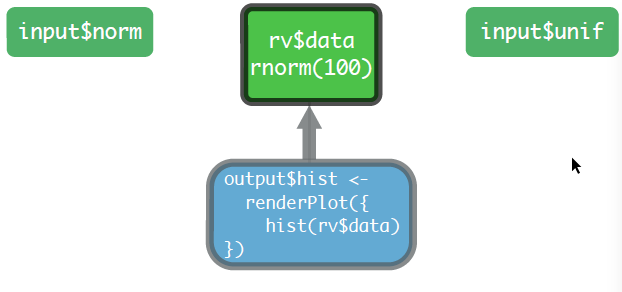
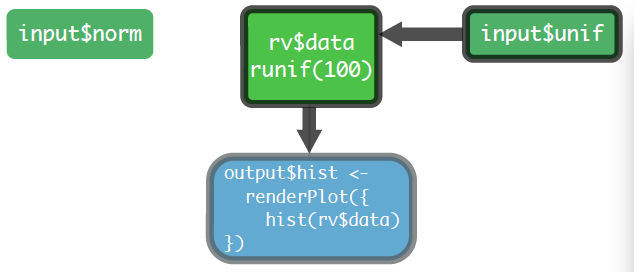
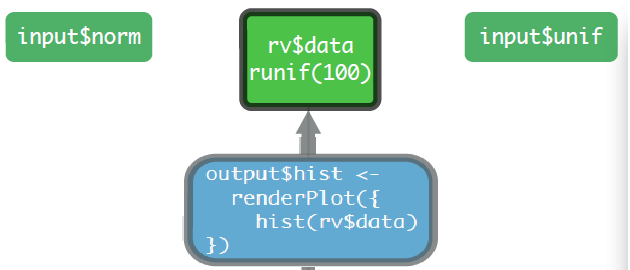
重點提示
- reactiveValues() creates a list of reactive values
- You can manipulate these values (usually with observeEvent())
小結
## Parting tips(非常重要!!!)
首先先看一下Shiny APP整個的架構。
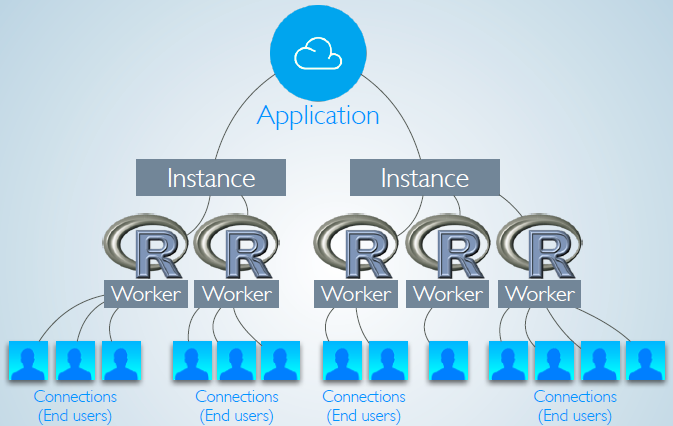
呼應前圖對應層級,有些的作業處理,就效能面是或是生效的範圍來說,就要放到對應的地方處理。舉例來說,在Server範圍以外的程式,將只被執行一次,某些特殊的初始作業,例如讀檔案存成df的作業,就是初始時,僅需處理一次的作業。
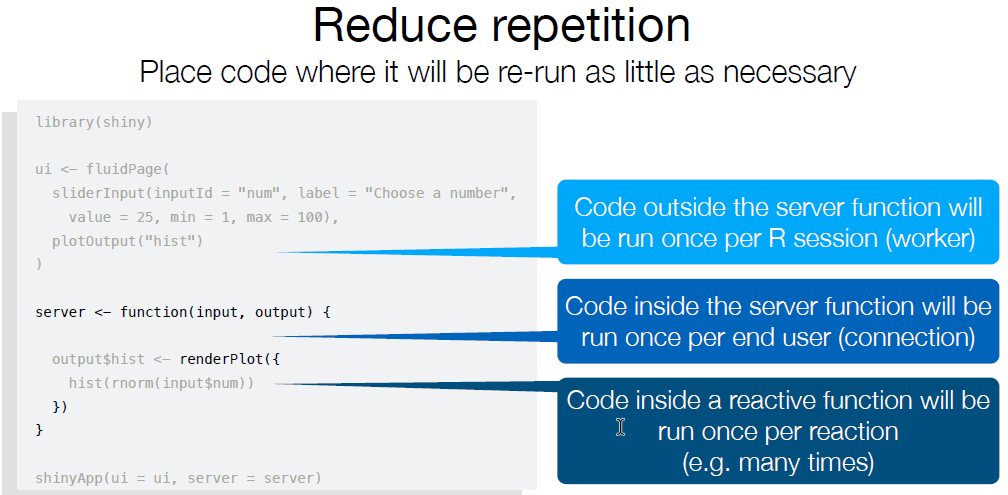
參考資料
How to start with Shiny – Part 2
[shiny-cheatsheet copy - RStudio](shiny-cheatsheet copy - RStudio)