Firebase 常被用於 App 與 網頁服務,
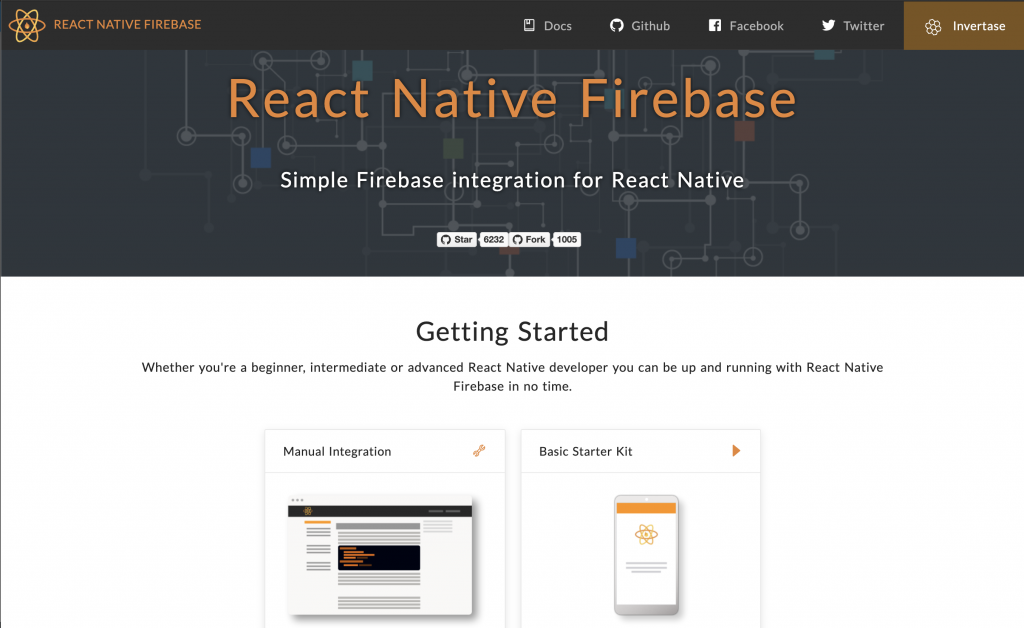
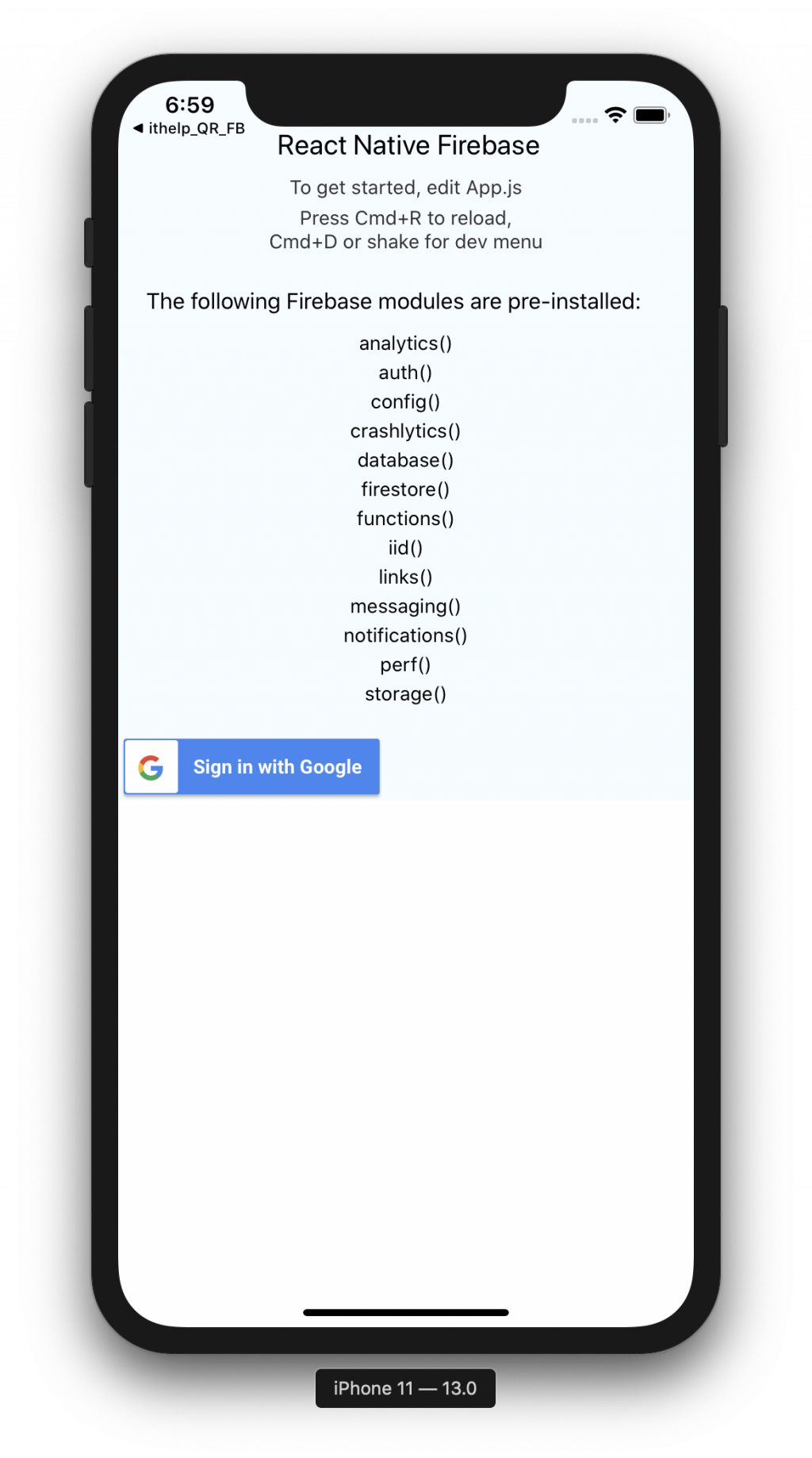
/* eslint-disable prettier/prettier */
import React from 'react';
import { StyleSheet, Platform, Image, Text, View, ScrollView, Alert } from 'react-native';
import firebase, { RemoteMessage } from 'react-native-firebase';
import AsyncStorage from '@react-native-community/async-storage';
import { GoogleSignin } from 'react-native-google-signin';
const iosConfig = {
};
const itHelpApp = firebase.initializeApp(iosConfig);
export default class App extends React.Component {
constructor() {
super();
this.state = {
isSigninInProgress: "",
isAuthenticated: false,
uid:"",
};
}
updata() {
let docID = "VQ255760627"
const ref = firebase.firestore().collection('testA').doc(docID);
itHelpApp.onReady().then((app) => {
app.firestore()
.runTransaction(async transaction => {
const doc = await transaction.get(ref);
// if it does not exist set the population to one
if (!doc.exists) {
transaction.set(ref, { content: "test" });
// return the new value so we know what the new population is
return null;
}
// exists already so lets increment it + 1
const newPopulation = doc.data().population + 4;
const newcontent = "VQ25576066"
transaction.update(ref, {
content: newcontent,
});
// return the new value so we know what the new population is
return newPopulation;
})
.then(newPopulation => {
console.log(
`Transaction successfully committed and new population is '${newPopulation}'.`
);
})
.catch(error => {
console.log('Transaction failed: ', error);
});
});
}
updataID(docID,fcmToken,alarmFcmToken) {
// let docID = "VQ255760627"
itHelpApp.onReady().then((app) => {
app.firestore().collection('users').doc(docID).set({
fcmTokens: firebase.firestore.FieldValue.arrayUnion(fcmToken),
alarmFcmTokens: firebase.firestore.FieldValue.arrayUnion(alarmFcmToken),
}).then(newPopulation => {
console.log(
`Transaction successfully committed and new population is '${newPopulation}'.`
);
})
.catch(error => {
console.log('Transaction failed: ', error);
});
});
}
async bootstrap() {
await GoogleSignin.configure({
scopes: ['https://www.googleapis.com/auth/drive.readonly'],
webClientId: '20749855338-pc4bbn60tijh4l1g5g8o54vh9fr09e0r.apps.googleusercontent.com', // required
// scopes: ['com.googleusercontent.apps.20749855338-pc4bbn60tijh4l1g5g8o54vh9fr09e0r'],
// webClientId: '20749855338-pc4bbn60tijh4l1g5g8o54vh9fr09e0r.apps.googleusercontent.com', // required
});
const { accessToken, idToken } = await GoogleSignin.signIn();
const credential = firebase.auth.GoogleAuthProvider.credential(idToken, accessToken);
await firebase.auth().signInWithCredential(credential);
}
// {"web":{"client_id":"802333868646-f5g03of7ff6lqikfkipgk59hlv44soee.apps.googleusercontent.com","project_id":"deliwater","auth_uri":"https://accounts.google.com/o/oauth2/auth","token_uri":"https://oauth2.googleapis.com/token","auth_provider_x509_cert_url":"https://www.googleapis.com/oauth2/v1/certs","client_secret":"yMJ5ip5mxYEnVGEboAi6blIo","redirect_uris":["https://deliwater.firebaseapp.com/__/auth/handler"],"javascript_origins":["http://localhost","http://localhost:5000","https://deliwater.firebaseapp.com"]}}
async componentDidMount() {
// TODO: You: Do firebase things
// const { user } = await firebase.auth().signInAnonymously();
// console.warn('User -> ', user.toJSON());
// this.Todos();
// const apnsToken = firebase.messaging().getAPNSToken();
// if (apnsToken) {
// console.log('User APNS Token:', apnsToken);
// }
firebase.auth().signInAnonymously()
.then((uidToken) => {
this.setState({
isAuthenticated: true,
uid:uidToken.user._user.uid,
});
console.log('User Token:', uidToken.user._user.uid,);
});
// this.bootstrap();
// console.log('User APNS Token:', firebase.messaging().getAPNSToken());
const fcmToken = firebase.messaging().getToken();
console.log('User fcm Token:', fcmToken);
// const uid = firebase.auth().currentUser.uid;
// const uid = firebase.auth().signInAnonymously();
// console.log('User uid:', itHelpApp.firebase.auth().currentUser.uid);
// await firebase.firestore().doc(`users/${this.state.uid}`)
// .update({
// fcmTokens: firebase.firestore.FieldValues.arrayUnion(fcmToken),
// }).then((response) => {
// console.log('auth', response, this.state.uid);
// return null;
// }).catch((error) => {
// console.log('Error auth:', error);
// });
const alarmFcmToken = await firebase.messaging().getToken(
firebase.app().options.messagingSenderId, // default to this app
'ALARM', // defaults to 'FCM'
);
console.log('User ALARM fcm Token:', alarmFcmToken);
this.updataID(this.state.uid,fcmToken,alarmFcmToken);
await itHelpApp.onReady().then((app) => {
app.firestore()
.doc(`users/${this.state.uid}`).update({
fcmTokens: firebase.firestore.FieldValue.arrayUnion(fcmToken),
alarmFcmTokens: firebase.firestore.FieldValue.arrayUnion(alarmFcmToken),
// firebase.firestore.FieldValue.X
}).then(newPopulation => {
console.log(
`update is OK '${newPopulation}'.`
);
})
.catch(error => {
console.log('update is failed: ', error);
});
});
this.updata();
this.checkPermission();
this.createNotificationListeners(); //add this line
this.messageListener = firebase.messaging().onMessage((message: RemoteMessage) => {
// Process your message as required
});
}
componentWillUnmount() {
this.notificationListener();
this.notificationOpenedListener();
this.messageListener();
}
//1
async checkPermission() {
const enabled = await firebase.messaging().hasPermission();
if (enabled) {
this.getToken();
} else {
this.requestPermission();
}
}
//2
async requestPermission() {
try {
await firebase.messaging().requestPermission();
// User has authorised
this.getToken();
} catch (error) {
// User has rejected permissions
console.log('permission rejected');
}
}
//3
async getToken() {
let fcmToken = await AsyncStorage.getItem('fcmToken');
if (!fcmToken) {
fcmToken = await firebase.messaging().getToken();
if (fcmToken) {
// user has a device token
await AsyncStorage.setItem('fcmToken', fcmToken);
}
}
}
async createNotificationListeners() {
/*
* Triggered when a particular notification has been received in foreground
* */
this.notificationListener = firebase.notifications().onNotification((notification) => {
const { title, body } = notification;
this.showAlert(title, body);
});
/*
* If your app is in background, you can listen for when a notification is clicked / tapped / opened as follows:
* */
this.notificationOpenedListener = firebase.notifications().onNotificationOpened((notificationOpen) => {
const { title, body } = notificationOpen.notification;
this.showAlert(title, body);
});
/*
* If your app is closed, you can check if it was opened by a notification being clicked / tapped / opened as follows:
* */
const notificationOpen = await firebase.notifications().getInitialNotification();
if (notificationOpen) {
const { title, body } = notificationOpen.notification;
this.showAlert(title, body);
}
/*
* Triggered for data only payload in foreground
* */
this.messageListener = firebase.messaging().onMessage((message) => {
//process data message
console.log(JSON.stringify(message));
});
}
showAlert(title, body) {
Alert.alert(
title, body,
[
{ text: 'OK', onPress: () => console.log('OK Pressed') },
],
{ cancelable: false },
);
}
// async googleLogin() {
// try {
// // add any configuration settings here:
// await GoogleSignin.configure();
// const data = await GoogleSignin.signIn();
// // create a new firebase credential with the token
// const credential = firebase.auth.GoogleAuthProvider.credential(data.idToken, data.accessToken)
// // login with credential
// const firebaseUserCredential = await firebase.auth().signInWithCredential(credential);
// console.warn(JSON.stringify(firebaseUserCredential.user.toJSON()));
// } catch (e) {
// console.error(e);
// }
// }
render() {
return (
<ScrollView>
<View style={styles.container}>
{/* <Image source={require('./assets/ReactNativeFirebase.png')} style={[styles.logo]} /> */}
<Text style={styles.welcome}>
Welcome to {'\n'} React Native Firebase
</Text>
<Text style={styles.instructions}>
To get started, edit App.js
</Text>
{Platform.OS === 'ios' ? (
<Text style={styles.instructions}>
Press Cmd+R to reload,{'\n'}
Cmd+D or shake for dev menu
</Text>
) : (
<Text style={styles.instructions}>
Double tap R on your keyboard to reload,{'\n'}
Cmd+M or shake for dev menu
</Text>
)}
<View style={styles.modules}>
<Text style={styles.modulesHeader}>The following Firebase modules are pre-installed:</Text>
{firebase.admob.nativeModuleExists && <Text style={styles.module}>admob()</Text>}
{firebase.analytics.nativeModuleExists && <Text style={styles.module}>analytics()</Text>}
{firebase.auth.nativeModuleExists && <Text style={styles.module}>auth()</Text>}
{/* https://rnfirebase.io/docs/v5.x.x/auth/social-auth */}
{firebase.config.nativeModuleExists && <Text style={styles.module}>config()</Text>}
{firebase.crashlytics.nativeModuleExists && <Text style={styles.module}>crashlytics()</Text>}
{firebase.database.nativeModuleExists && <Text style={styles.module}>database()</Text>}
{firebase.firestore.nativeModuleExists && <Text style={styles.module}>firestore()</Text>}
{firebase.functions.nativeModuleExists && <Text style={styles.module}>functions()</Text>}
{firebase.iid.nativeModuleExists && <Text style={styles.module}>iid()</Text>}
{firebase.links.nativeModuleExists && <Text style={styles.module}>links()</Text>}
{firebase.messaging.nativeModuleExists && <Text style={styles.module}>messaging()</Text>}
{firebase.notifications.nativeModuleExists && <Text style={styles.module}>notifications()</Text>}
{firebase.perf.nativeModuleExists && <Text style={styles.module}>perf()</Text>}
{firebase.storage.nativeModuleExists && <Text style={styles.module}>storage()</Text>}
</View>
{/* <GoogleSigninButton
style={{ width: 192, height: 48 }}
size={GoogleSigninButton.Size.Wide}
color={GoogleSigninButton.Color.Dark}
onPress={this.googleLogin}
disabled={this.state.isSigninInProgress} /> */}
{/* {this.Todos()} */}
</View>
</ScrollView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
// alignItems: 'center',
backgroundColor: '#F5FCFF',
},
logo: {
height: 120,
marginBottom: 16,
marginTop: 64,
padding: 10,
width: 135,
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
modules: {
margin: 20,
},
modulesHeader: {
fontSize: 16,
marginBottom: 8,
},
module: {
fontSize: 14,
marginTop: 4,
textAlign: 'center',
}
});